Shell Scripting with Practical Examples
Shell scripting is a vital tool for automating tasks in Linux and Unix-like systems. It allows you to streamline processes, manage system operations, and boost productivity.

In this blog, I have covered key shell scripting topics with practical examples and clear explanations.
Key Components of Shell Script:
Shebang (#!/bin/bash
)
The shebang is the first line in a script and specifies the interpreter that should be used to execute the script.

Example:
#!/bin/bash
echo "Hello, World!"
Explanation:
This script uses #!/bin/bash
to indicate that the Bash shell should be used to execute the script. The echo
command prints "Hello, World!" to the terminal.
Variables:
Variables in shell scripting are used to store and manipulate data. They can hold values such as strings, integers, and the results of commands.
Example:
#!/bin/bash
name="Maha Lakshmi"
echo "Hello, $name!"
Explanation:
The variable name
stores the value "Maha Lakshmi," and the echo
command uses $name
to print "Hello, Maha Lakshmi!".
we have some special variables in shell scripting
- `$0` — Script Name: Represents the name of the script being executed.
Example:
#!/bin/bash
echo "The script name is: $0"
Explanation:
If the script is named `myscript.sh`, running it would output “The script name is: myscript.sh”.
2. `$#` — Number of Arguments: Represents the number of positional parameters (arguments) passed to the script.
Example:
#!/bin/bash
echo "Number of arguments passed: $#"
Explanation:
If you run `./myscript.sh arg1 arg2`, it will output “Number of arguments passed: 2”.
3. `$@` — All Arguments as Separate Strings: Represents all the arguments passed to the script as separate words.
Example:
#!/bin/bash
for arg in "$@"
do
echo "Argument: $arg"
done
Explanation:
If you run `./myscript.sh hello world`, it will output “Argument: hello” and “Argument: world” on separate lines.
4. `$*` — All Arguments as a Single String: Represents all the arguments passed to the script as a single string.
Example:
#!/bin/bash
echo "All arguments as a single string: $*"
Explanation:
If you run `./myscript.sh hello world`, it will output “All arguments as a single string: hello world”.
5. `$?` — Exit Status: Represents the exit status of the last command executed. A value of `0` typically indicates success, while any non-zero value indicates an error.
Example:
#!/bin/bash
ls -lsls
echo "Exit status of the last command: $?"
Explanation:
Since ls -lsls
` doesn’t exist, the `ls` command will fail, and the script will output “Exit status of the last command: 2” (or another non-zero value). Where exit status 0 indicates success.
6. `$$` — Process ID (PID): Represents the process ID of the current shell script.
Example:
#!/bin/bash
echo "The process ID of this script is: $$"
Explanation:
This will print the process ID of the script, which is useful for managing script execution.
7. `$!` — PID of Last Background Command: Represents the process ID of the last command executed in the background.
Example:
#!/bin/bash
sleep 30 &
echo "PID of the last background command: $!"
Explanation:
This will print the process ID of the `sleep 30` command running in the background.
Arrays:
Arrays store multiple values in a single variable, allowing you to manage lists of data efficiently in your scripts.
Example:
#!/bin/bash
# Define an array
fruits=("Apple" "Banana" "Cherry")
# Access array elements
echo "First fruit: ${fruits[0]}"
echo "All fruits: ${fruits[@]}"
Explanation:
Thefruits
array contains three elements: "Apple," "Banana," and "Cherry." The${fruits[0]}
accesses the first element and${fruits[@]}
all elements.
Conditional Statements:
Conditional statements allow you to make decisions in your script based on certain conditions. The if-else
statement is commonly used to execute different blocks of code based on the evaluation of a condition.
Example:
#!/bin/bash
num=5
if [ $num -gt 10 ]; then
echo "Number is greater than 10"
else
echo "Number is 10 or less."
fi
Explanation:
This script checks if the variable num
is greater than 10. If it is, it prints "Number is greater than 10"; otherwise, it prints "Number is 10 or less.".
Loops:
Loops are used to execute a block of code repeatedly.
Example:
#!/bin/bash
for i in {1..5}; do
echo "Iteration $i"
done
Explanation:
This for
loop iterates five times, printing "Iteration 1" to "Iteration 5".
Functions:
Functions in shell scripting allow you to encapsulate and reuse code blocks. Functions can take arguments and return values, making your scripts more modular and maintainable.
Example:
#!/bin/bash
greet() {
echo "Hello, $1!"
}
greet "Maha"
Explanation:
The greet
function takes an argument ($1
) and prints a greeting. When called with "Maha", it prints "Hello, Maha!".
User Input:
Shell scripts can interact with users by accepting input during execution using the read
command.
Example:
#!/bin/bash
echo "Enter your name:"
read name
echo "Hello, $name!"
Explanation:
The script prompts the user to enter their name and then greets them using the echo
command.
File Operations:
Shell scripts often involve file manipulation, such as reading from or writing to files. The cat
, echo
, and >
operators are frequently used for these tasks.
Example:
#!/bin/bash
echo "Content of file.txt:"
cat file.txt
echo "Lines containing 'error':"
grep 'error' file.txt
echo "Replacing 'old' with 'new' in file.txt:"
sed -i 's/old/new/g' file.txt
Explanation:
This script displays the content of file.txt
, searches for lines containing "error," and replaces occurrences of "old" with "new" in the file.
Error Handling:
It’s essential to handle errors gracefully to prevent scripts from failing unexpectedly.
Example:
#!/bin/bash
set -e
cp source.txt destination.txt
echo "File copied successfully"
Explanation:
The set -e
command makes the script exit immediately if any command fails. If the cp
command fails, the script will terminate without running the echo
command.
Script Debugging
Debugging helps you identify and fix errors in your scripts.
Example:
#!/bin/bash
# Enable debugging
set -x
# Example commands
echo "Debugging mode enabled"
sum=$(($1 + $2))
echo "Sum: $sum"
Explanation:
`set -x` enables debugging, showing each command and its result.
Command Substitution:
Command substitution allows you to capture the output of a command and use it as a variable or within another command.
Example:
#!/bin/bash
# Get the current date
current_date=$(date)
# Use the date in a message
echo "Today's date is: $current_date"
Explanation:
The $(date)
syntax captures the output of the date
command.
The captured data is stored in the current_date
variable and used in the echo
statement.
Benefits of Shell Scripting:
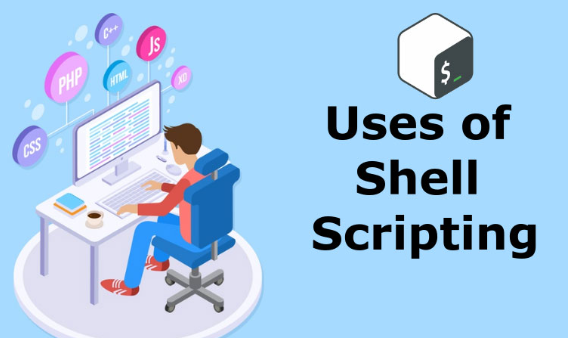
- Automation: Automate repetitive tasks, reducing manual effort and the potential for human error.
- Efficiency: Combine multiple commands and processes into a single script to perform complex operations quickly.
- Flexibility: Easily adapt scripts to handle various scenarios and system configurations.
- Portability: Scripts written in shell are generally portable across different Linux-like systems, making them useful in diverse environments.
Conclusion:
Shell scripting is essential for automating tasks in Linux and Unix-like systems. By understanding key concepts such as variables, special variables, arrays, conditional statements, loops, functions, and file operations, you can create powerful and efficient scripts. Mastering these elements will help you streamline processes, handle errors gracefully, and debug effectively, enhancing your productivity and system management.
Success usually comes to those who are too busy to be looking for it. — Henry David Thoreau
Keep learning!!!✨